Image resizing tool for your e-commerce or social media website
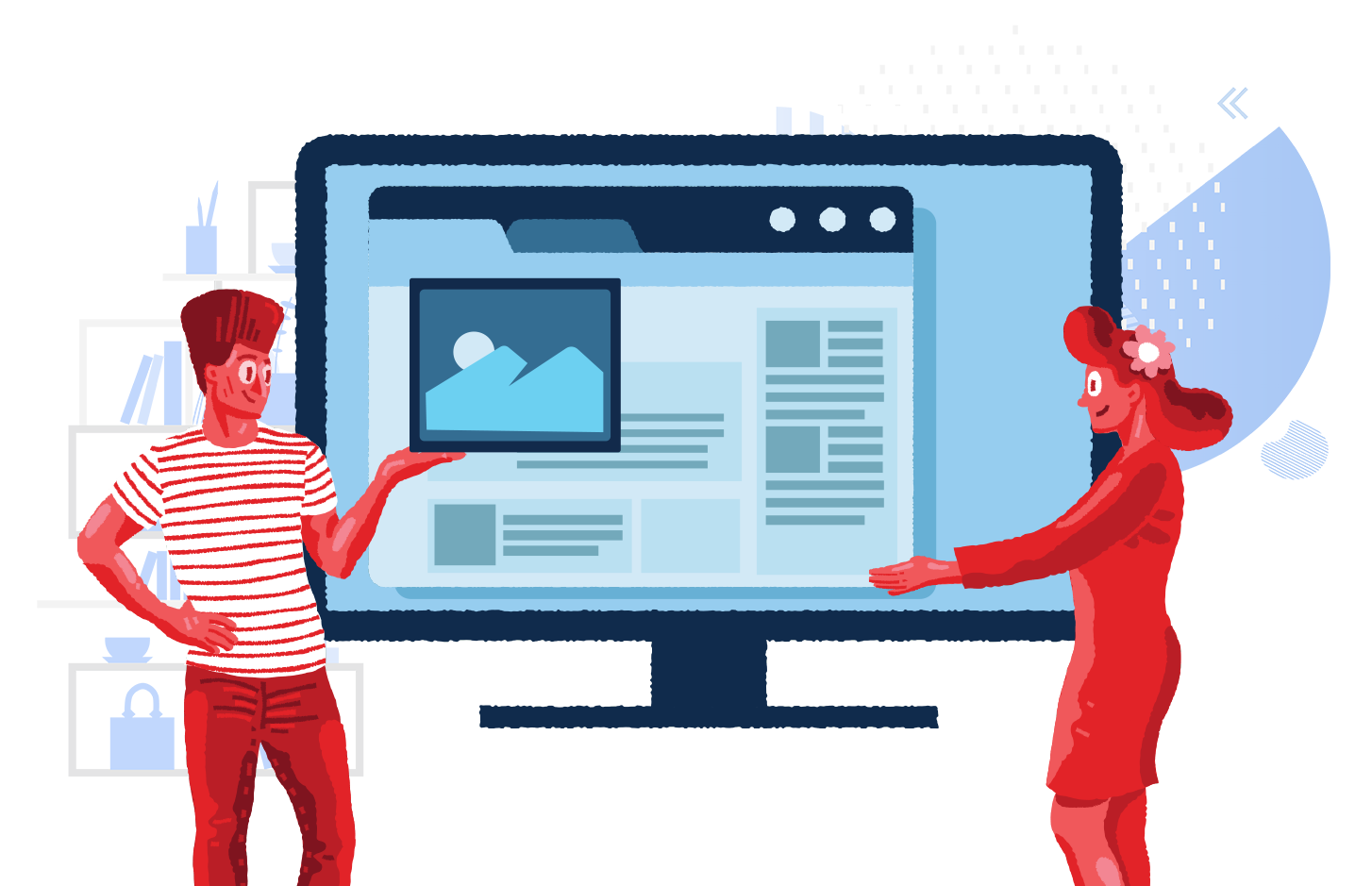
A developer can easily create a workflow using the tweekit web application to crop the headshots for all HR employee photos from any format uploaded, making it easy to get the content you need, with no hassles from your employees.
Before starting, always include the
tweekit_bundle.js
-
Rest Version
-
Widget Version
rest_api_example.js
const fetch = require('node-fetch');
const headers = {
"apikey": "{Example Key}",
"apisecret": "{Example Secret}",
"Content-Type": "application/json;charset=UTF-8"
};
async function upload({ file, filename }) {
formData.append("name", filename);
formData.append("file", fs.createReadStream(file));
const fetchUrl = `${baseUrl}/image/upload`;
const response = await fetch(fetchUrl, opts);
const docID = response.headers.get('x-tweekit-docid')
return { docID, response }
}
async function resize({ docID, width, height }) {
const format = 'png'
const fetchUrl = `${baseUrl}/image/${docID}`;
const options ={
headers ,
method: 'POST',
body: JSON.stringify({
height,
width,
fmt: format
})
};
const response = await fetch(fetchUrl,options);
return response.body;
}
async function resizeImg({ imgUrl, width, height, filename}) {
const imgFile = new File(imgUrl)
// upload image to server
const { docID } = await upload({ file: imgFile, filename })
const imgBlob = await resize({
docID,
width: parseInt(width),
height: parseInt(height)
})
const newImgUrl = URL.createObjectURL(imgBlob)
return newImgUrl
}
$(document).ready(function() {
$('.lazyImg').each(async function() {
const {
imgUrl,
width ,
height ,
filename
} = $(this).data()
const croppedImgUrl = await resizeImg({imgUrl,width, height, filename})
$(this).attr('src', croppedImgUrl)
})
})